Python Tutorial
Simple to start, powerful to grow.
1. Introduction to Python
- Python is an interpreted, high-level programming language.
- It emphasizes readability and simplicity.
- Common uses: Web development (Django, Flask), Data Science, Automation, and Scripting.
2. Setting Up Python
- Download Python:
- Install an IDE:
- Use IDLE (comes with Python) or install VS Code, PyCharm, or Jupyter Notebook.
3. Writing Your First Python Program
Open a Python file (e.g., example.py
) and write:

Run the program:
- In the terminal:
python example.py
.
4. Variables and Data Types
- Variables store data values.
- Python is dynamically typed, meaning you don’t need to declare the type.
Example:

5. Operators
Python supports several types of operators:
- Arithmetic Operators:
+
, -
, *
, /
, **
(power), %
(modulus) - Comparison Operators:
==
, !=
, >
, =
, - Logical Operators:
and
, or
, not
Example:

6. Conditional Statements
Control the flow of your program using if
, elif
, and else
.
Example:
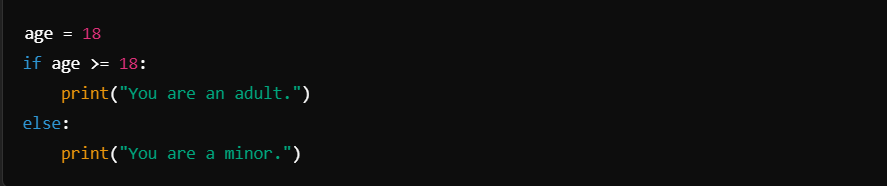
7. Loops
Loops allow you to repeat a block of code.
For Loop:

While Loop:

8. Functions
Functions are reusable blocks of code.
Example:

9. Lists and Dictionaries
Lists:
- Ordered collection of items.

Dictionaries:

10. File Handling
Python makes it easy to work with files.
Write to a File:

Read from a File:

11. Practice Examples
- Simple Calculator:
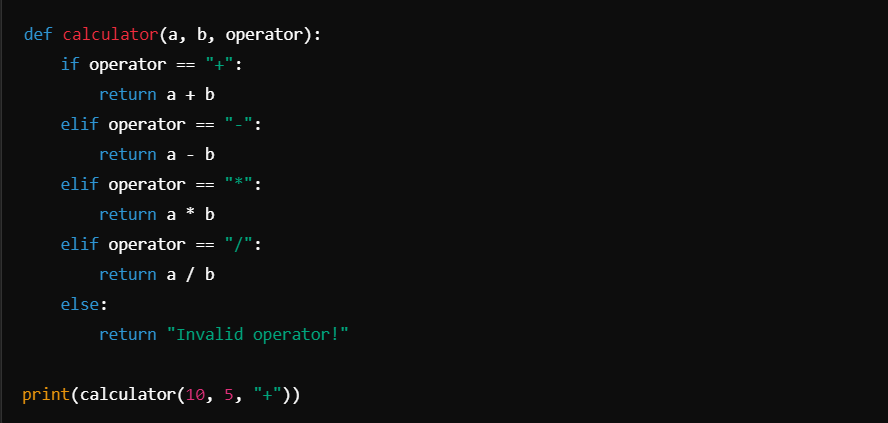
Guess the Number Game:
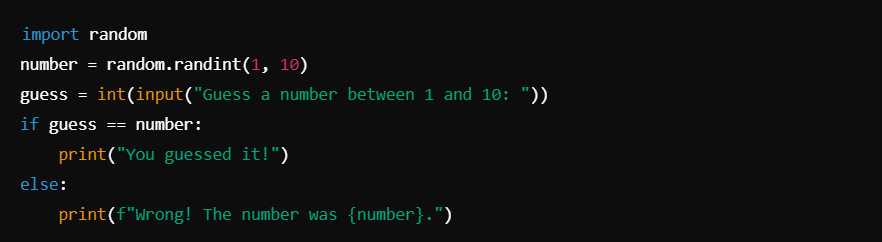
Next Steps
- Practice coding small projects (e.g., To-Do App, Mini Games).
- Learn advanced topics like:
- Object-Oriented Programming (OOP)
- Modules and Packages
- Libraries like NumPy and Pandas.